10 Essential Coding Best Practices For React Native Devs
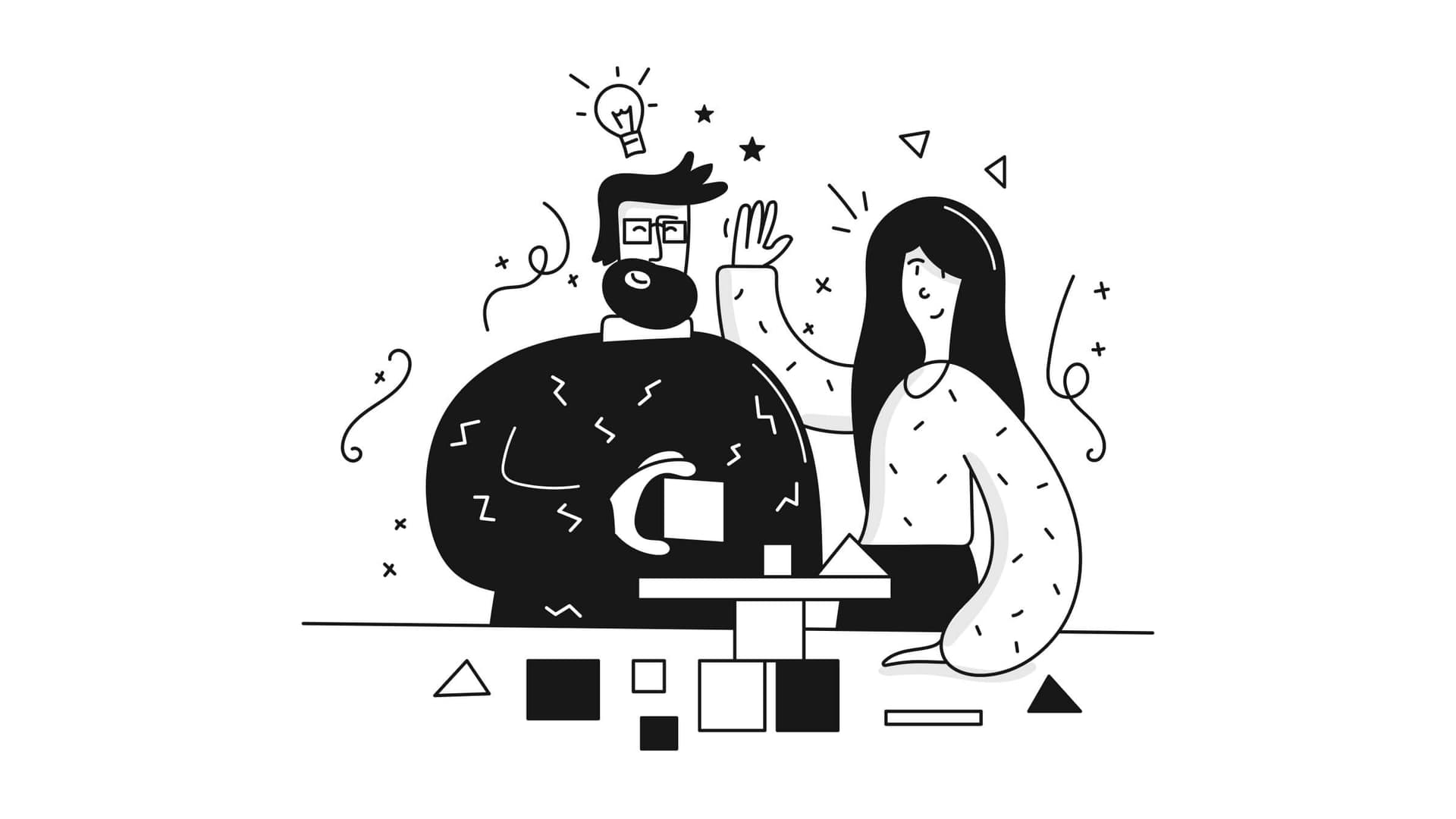
React Native is a great framework for building cross-platform mobile apps, especially when combined with Expo. However, it can be challenging to know what the best practices are for React Native development. In this article, we will discuss some of the most important best practices that every React Native developer should follow.
Introduction
In this article, we will discuss the best practices for React Native / Expo developers. We will cover the following topics:
- Hooks' Based Approach
- Update to latest SDK
- Expo Router instead of React Navigation
- Naming Conventions
- Consistent Styling
- Consistent Imports
- Image Optimization
- FlashList instead of FlatList
- Use AsyncStorage for Local Storage
- Build a deployment approach (CI/CD)
Hooks' Based Approach
React Hooks have revolutionized the way we write React components, providing a more concise and readable code structure. When developing with React Native or Expo, embracing a Hooks-based approach is highly recommended. Hooks allow us to manage state and side effects without relying on class components. This makes it easier to write reusable code that can be shared across different projects.
Update to latest SDK
Most of the time, updating to the latest SDK is a good idea. By doing so, you will be able of benefiting from:
- New features and bug fixes that are not available in older versions of the SDK.
- Improved performance and stability.
- Better compatibility with third-party libraries.
- Your app will be more future-proof, and can be easily deployed onto the stores.
But also keep in mind, that updating to the latest SDK can sometimes break your app. So, it's always a good idea to test your app thoroughly before deploying it to production.
Expo Router instead of React Navigation
Expo Router is the new approach to navigation in Expo apps, it uses a file-based approach to navigation. This means that when creating a new file, you are also creating a new route. This makes it easier to manage your routes and keep them organized. It also makes it easier to add new routes without having to worry about breaking existing ones. And recently the version 2 of Expo Router has been released, which is a major improvement over the previous version. It includes a lot of new features and improvements, such as:
- Universal Linking
- Automatic TypeScript
- Static Routes
- Async Routes
You can read more about it here.
Naming Conventions
This is a must when working on a team project. It's important to have a consistent naming convention across the codebase. This will make it easier for other developers to understand your code and contribute to the project. It will also make it easier for you to maintain the codebase in the future.
Consistent Styling
It's important to have a consistent styling across the codebase. I personally use Tailwind CSS along with React Native Paper because it's easy to use and it provides a lot of useful components that can be used in different projects. It also has a lot of built-in styles that can be used to style your components. But what I'm trying to say is that you should use whatever works best for you and your team : A consistent styling approach will make it easier for other developers to understand your code and contribute to the project.
Consistent Imports
It's important to have a consistent import approach across the codebase. This will make it easier for other developers to understand your code and contribute to the project. It will also make it easier for you to maintain the codebase in the future.
Image Optimization
In React Native apps, images can sometimes cause your app to slow down. To make sure your app runs smoothly, it's important to optimize your images specifically for mobile devices. This involves adjusting their size to fit the screen, reducing their file size through compression, and using the right file format that works best for mobile devices. By taking these steps, you can ensure that your app's images load quickly and contribute to a faster overall user experience.
FlashList instead of FlatList
FlashList is a new component that was recently introduced in React Native. It's a replacement for the FlatList component, which is used to render lists of data. The main difference between the two is that FlashList uses a virtualized approach to rendering lists, which means that it only renders the items that are currently visible on the screen. This makes it more performant than FlatList, especially when dealing with large lists of data.
You can check it out here
Use AsyncStorage for Local Storage
AsyncStorage is a new API that was recently introduced in React Native. It's a replacement for the AsyncStorage API, which is used to store data locally on the device. The main difference between the two is that AsyncStorage uses a more efficient approach to storing data, which means that it's faster and more performant than AsyncStorage. It also has a lot of built-in features that make it easier to work with, such as automatic serialization and deserialization of data.
You can check it out here
Build a deployment approach (CI/CD)
Of course building a CI/CD approach is not a must, but it's highly recommended. It will make it easier for you to deploy your app to the stores and keep it up-to-date with the latest changes. It will also make it easier for other developers to contribute to the project in the future. You can use Github Actions or any other CI/CD tool that works best for you and your team. You can also use Expo Updates to deploy your app to the stores.
You can check out Expo Updates here. I highly recommend it because it makes it easier to deploy your app to the stores and keep it up-to-date with the latest changes.
Conclusion
By using these best practices, you can ensure that your React Native / Expo app is as performant and maintainable as possible. You can work at ease knowing that your codebase is clean and well-organized, which will make it easier for other developers to contribute to the project in the future. Even collaborating with other developers will be easier because they won't have to spend time figuring out how things work or where things are located within the codebase.