Some useful React / React Native libraries that you must know in 2023
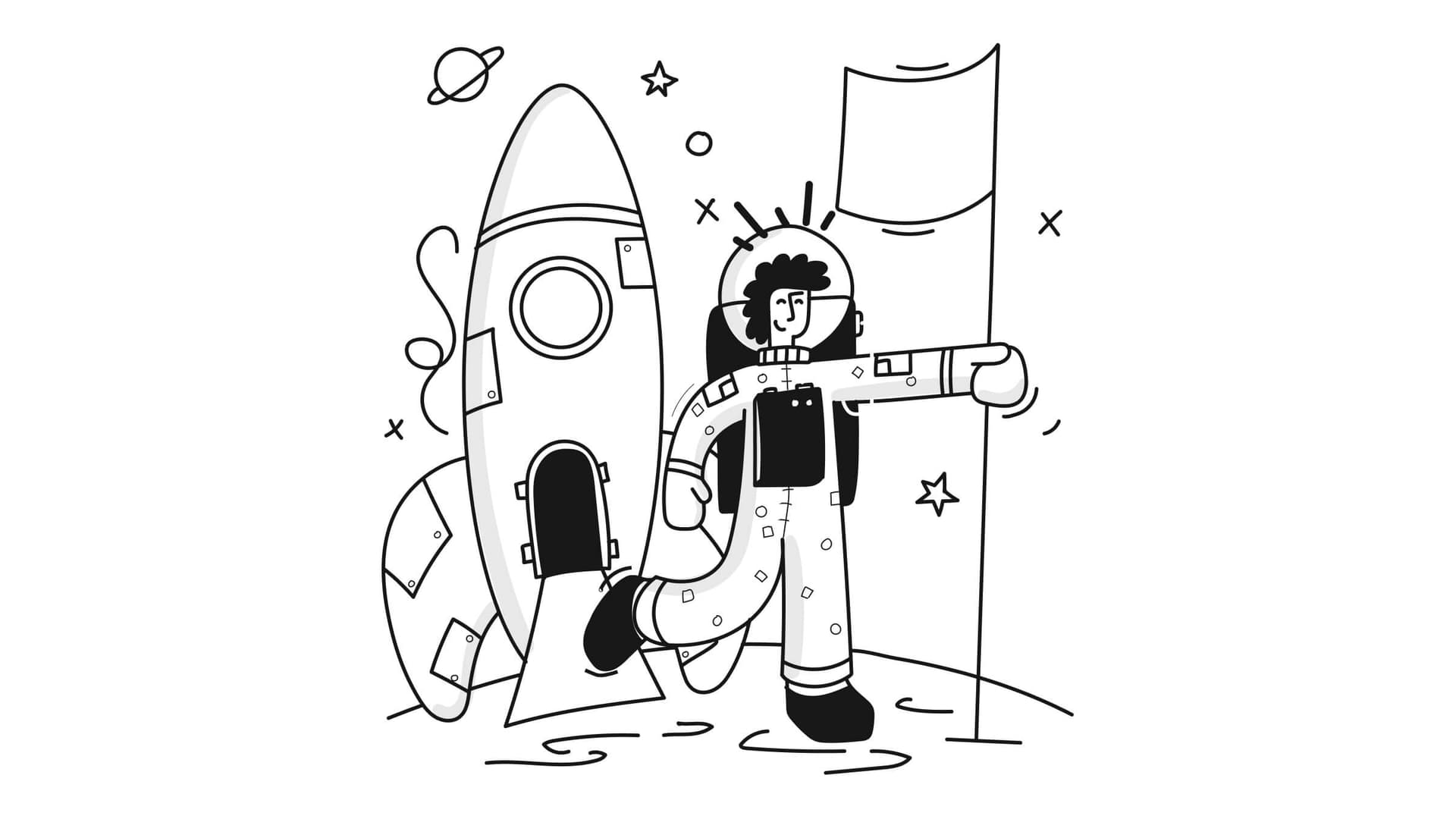
In this article, we will see some useful libraries that you must know in 2023 in the React / React Native ecosystem.
React is the most trending library in the JavaScript ecosystem. It is used to build user interfaces (UI) and is maintained by Facebook. React Native on the other hand is a framework that allows you to build mobile applications using React. I use it with Expo personally. And it makes me very productive. React / React Native have a large community and a lot of libraries are created every day. In this article, we will see some useful libraries that you must know in 2023.
Zod
Zod has been gaining a lot of attention lately. It is a TypeScript-first schema declaration and validation library. It is very easy to use and has a lot of features. It is also very fast and has a small bundle size.
Here is a table comparison between Zod and other libraries (I like to use tables 😅):
Library | Bundle size | Validation | TypeScript support | Customizable | Asynchronous support | Rich Validation Rules |
---|---|---|---|---|---|---|
Zod | Small | Yes | Yes | Yes | Yes | Yes |
Yup | Small | Yes | Yes | Yes | Yes | Yes |
Joi | Medium | Yes | Yes | Yes | Yes | Yes |
Validator | Small | Yes | Yes | Yes | No | No |
Zod has a very powerfull API. Here is an example:
import { z } from "zod"
// This is a schema to validate an object
// The object must have a name property of type string with a minimum length of 3 and a maximum length of 10
// The object must have an age property of type number that is an integer and positive
const schema = z.object({
name: z.string().min(3).max(10),
age: z.number().int().positive(),
})
// This is a schema to validate an array
// The array must have a minimum length of 1 and a maximum length of 10
// The array must contain only strings
const schema = z.array(z.string()).min(1).max(10)
Zod is very easy to use and has a lot of features. I recommend you to use it in your next project.
React Query
React Query is a library that allows you to fetch, cache and update data in your React applications. Everybody knows that fetching data in React is not easy. You have to deal with a lot of things like loading, error, caching, etc. React Query lift this burden from you and allows you to focus on your business logic.
Here is an example:
import { useQuery } from "react-query"
function App() {
const { isLoading, error, data } = useQuery("repoData", () =>
fetch("https://api.github.com/repos/tannerlinsley/react-query").then(
(res) => res.json()
)
)
if (isLoading) return "Loading..."
if (error) return "An error has occurred: " + error.message
return (
<div>
<h1>{data.name}</h1>
<p>{data.description}</p>
<strong>👀 {data.subscribers_count}</strong>{" "}
<strong>✨ {data.stargazers_count}</strong>{" "}
<strong>🍴 {data.forks_count}</strong>
</div>
)
}
In this example, we are fetching data from the GitHub API. React Query will handle the loading and error states for us. It will also cache the data for us. So if we refetch the data, it will not make a new request to the API. It will return the cached data.
Here is a set of other features that React Query provides:
- Pagination : React Query provides a
useInfiniteQuery
hook that allows you to fetch data in pages. - Infinite loading : React Query provides a
useInfiniteQuery
hook that allows you to fetch data infinitely. - Mutations : React Query provides a
useMutation
hook that allows you to mutate data. - Refetching : React Query provides a
refetch
function that allows you to refetch data. - Polling : React Query provides a
refetch
function that allows you to refetch data periodically. - And more...
Zustand
I tired of using the Redux library. It is very complex and has a lot of boilerplate. I was looking for a library that is easy to use and has a small bundle size. Zustand is a great alternative for a state management library. It is very easy to use and very small and has a lot of features.
Here is a comparison between Zustand and Redux (Or Redux Toolkit):
import create from "zustand"
// Zustand
const useStore = create((set) => ({
count: 1,
inc: () => set((state) => ({ count: state.count + 1 })),
dec: () => set((state) => ({ count: state.count - 1 })),
}))
// Redux
const initialState = { count: 1 }
function reducer(state = initialState, action) {
switch (action.type) {
case "INCREMENT":
return { count: state.count + 1 }
case "DECREMENT":
return { count: state.count - 1 }
default:
return state
}
}
const store = createStore(reducer)
store.dispatch({ type: "INCREMENT" })
store.dispatch({ type: "DECREMENT" })
As you can see Zustand is very easy to use. It is also very small. It has a bundle size of 1.5 KB. Other people prefer Jotai over Zustand. Jotai is also a great alternative for a state management library. It is very small and has a bundle size of 1.2 KB. They are made by the same author so why not try them both and see which one you prefer.
React Native Paper
Let's hop into the React Native / Expo ecosystem. React Native Paper is a library that provides you with a set of components that follow the Material Design guidelines. I, personally, have gotten accustomed to the Flutter ecosystem. I like the Material Design guidelines and I like to use them in my React Native applications. So I think this is a great library to use. I also use it along Tailwind CSS to style my applications, which I will talk about later.
Here is an example:
import { Appbar } from "react-native-paper"
const MyComponent = () => (
<Appbar.Header>
<Appbar.BackAction onPress={() => {}} />
<Appbar.Content title="Title" subtitle="Subtitle" />
<Appbar.Action icon="magnify" onPress={() => {}} />
<Appbar.Action icon="dots-vertical" onPress={() => {}} />
</Appbar.Header>
)
export default MyComponent
Clean and neet. Damn, I like it.
Use Hooks
Use Hooks is a website that provides you with a set of React hooks that you can use in your applications.
A collection of modern, server-safe React hooks - from the ui.dev team, as they say.
I recommend using it so that you don't have to reinvent the wheel.
Expo Updates
I would really thank the Expo team for this feature. I reserved a special section for it. Expo Updates is a feature that allows you to update your application without going through the App Store or the Play Store.
Although I found it a little bit hard to use at first, I think it is a great feature. So why not?
Tailwind CSS / Native Wind
Who doesn't use it these days? Tailwind CSS is a utility-first CSS framework. It is very easy to use and has a lot of features. It is also very customizable and can be used in mobile applications as well (using the Native Wind library).
Here is an example:
import { Text, View } from "react-native"
const MyComponent = () => (
<View className="flex flex-col items-center justify-center h-screen">
<Text className="text-2xl font-bold">Hello World!</Text>
</View>
)
export default MyComponent
Conclusion
I hope you liked this article. I will try to update it as much as I can. I will also try to add more libraries to it. If you have any suggestions, please let me know.